TensorSpace Layer has two status open and close,
default to "close". We can set layer's initial status before model is initialized
and change layer's status after model is initialized.
Set Initial Status
There are two ways to set initial status for TensorSpace Layer, set status in TensorSpace model or in a
specific layer.
Set in Model
Configure TensorSpace model's layerInitStatus attribute, open or close, default to "close".
"close" to show all layers as an aggregation, "open" to show all layers as expanded feature maps.
let model = new TSP.models.Sequential( container, {
// or "close"
layerInitStatus: "open"
} );
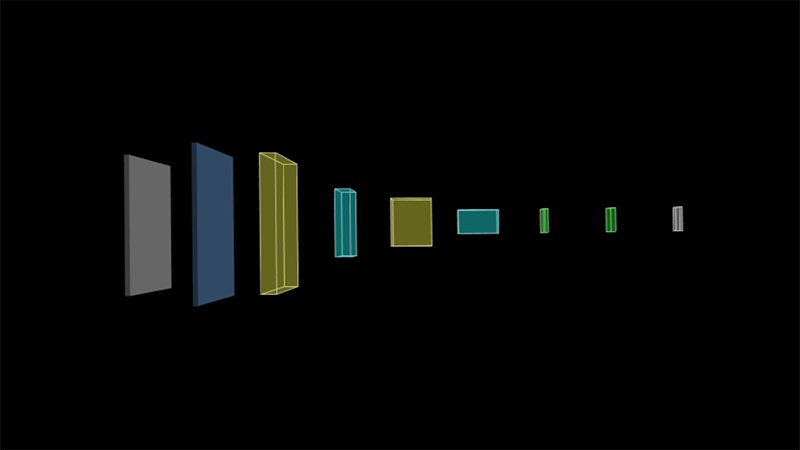
Fig. 1 - Initialize all layers with "close" status
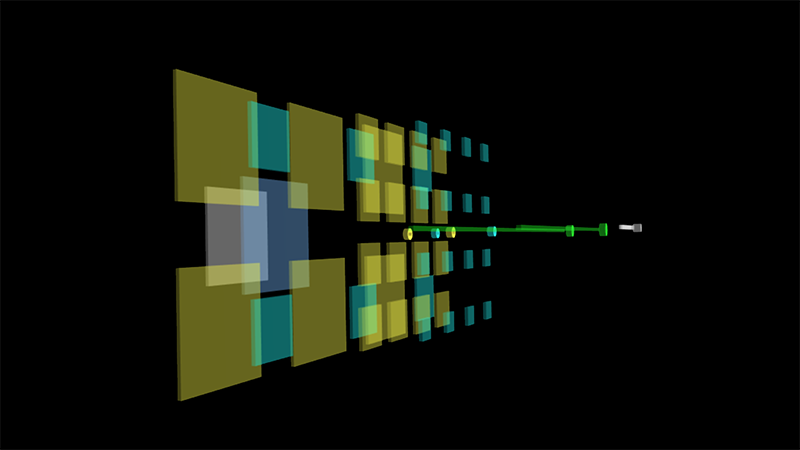
Fig. 2 - Initialize all layers with "open" status
Set in Specific Layer
Configure TensorSpace Layer's initStatus attribute, open or close, default to "close", if
set, this attribute will override model's layerInitStatus, which means
that model's layerInitStatus will not take effect to this specific Layer.
let conv2dLayer = new TSP.layers.Conv2d({
// or "close"
initStatus: "open"
});
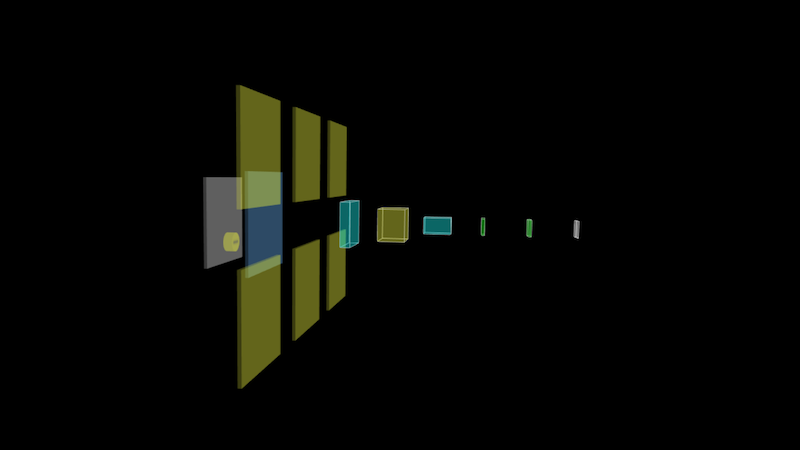
Fig. 3 - Set a specific Layer's initStatus to be open
Change Status
There are two ways to change layer's status after TensorSpace model is initialized, click layer elements in
3D scene or use APIs (.openLayer() & .closeLayer()) to control status.
Click Layer elements
Click the layer aggregation to open Layer in the 3D scene.
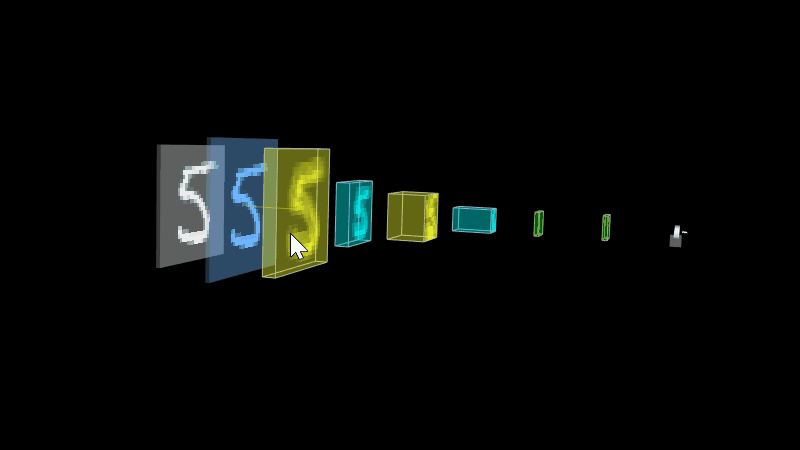
Fig. 4 - Click Conv2d Layer aggregation to open layer
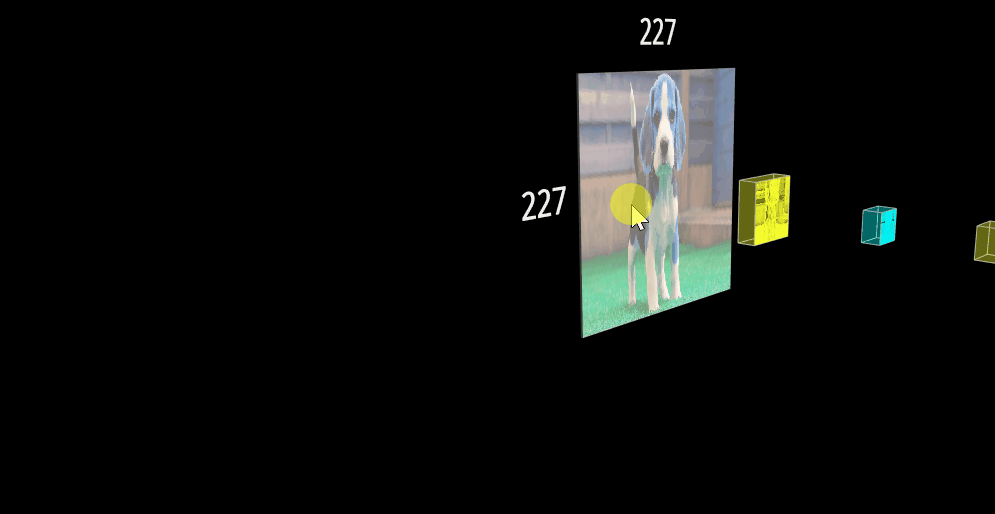
Fig. 5 - Click RGBInput Layer aggregation to open layer
Click the close button to close Layer in the 3D scene.
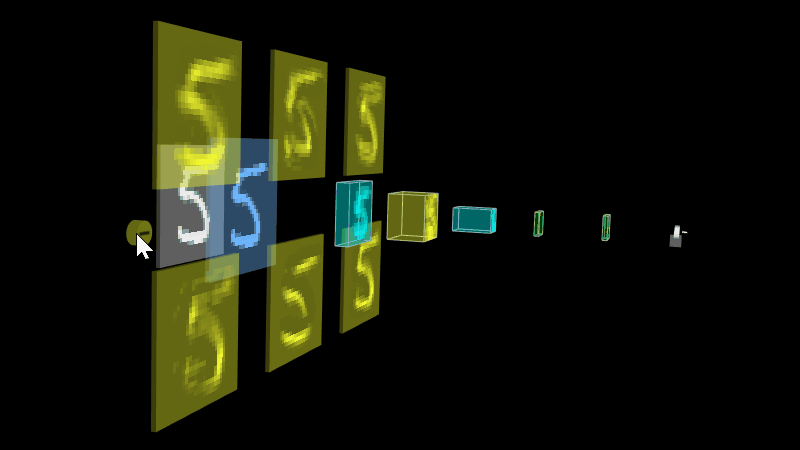
Fig. 6 - Click close button to close Conv2d Layer
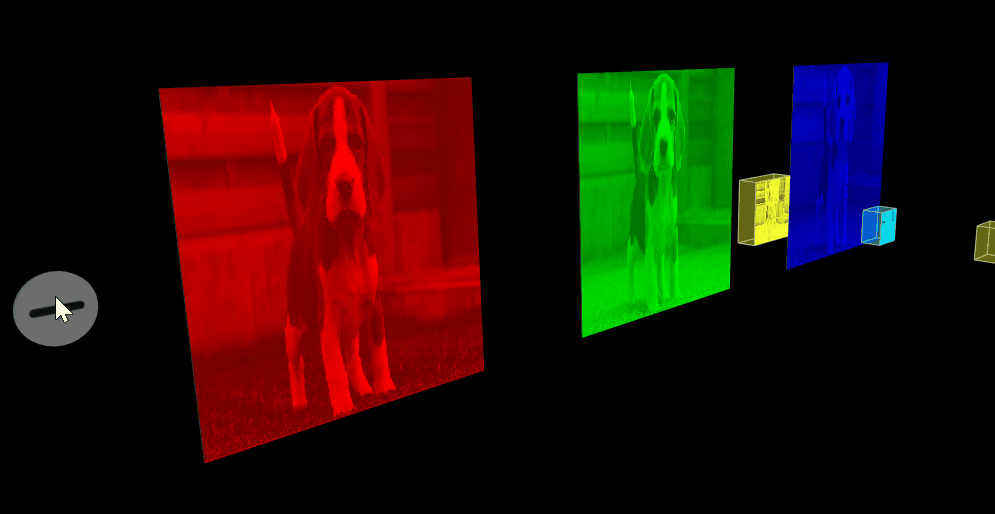
Fig. 7 - Click close button to close RGBInput Layer
Control APIs
Use .openLayer() API to open Layer, if layer is already in "open"
status, the layer will keep open.
let conv2dLayer = new TSP.layers.Conv2d( config );
model.add( conv2dLayer );
// ... add more layers for model.
model.init();
// Call openLayer API to open layer.
conv2dLayer.openLayer();
Use .closeLayer() API to close Layer, if layer is already in "close"
status, the layer will keep close.
let conv2dLayer = new TSP.layers.Conv2d( config );
model.add( conv2dLayer );
// ... add more layers for model.
model.init();
// Call closeLayer API to close layer.
conv2dLayer.closeLayer();