The "Load" of TensorSpace is the process to read and store the pre-trained ML model in the TensorSpace
model, which makes it possible to use the inference results from the pre-trained network by providing the
input.
The functionality of "Load" is realized by calling the .load() method
of the constructed model object.
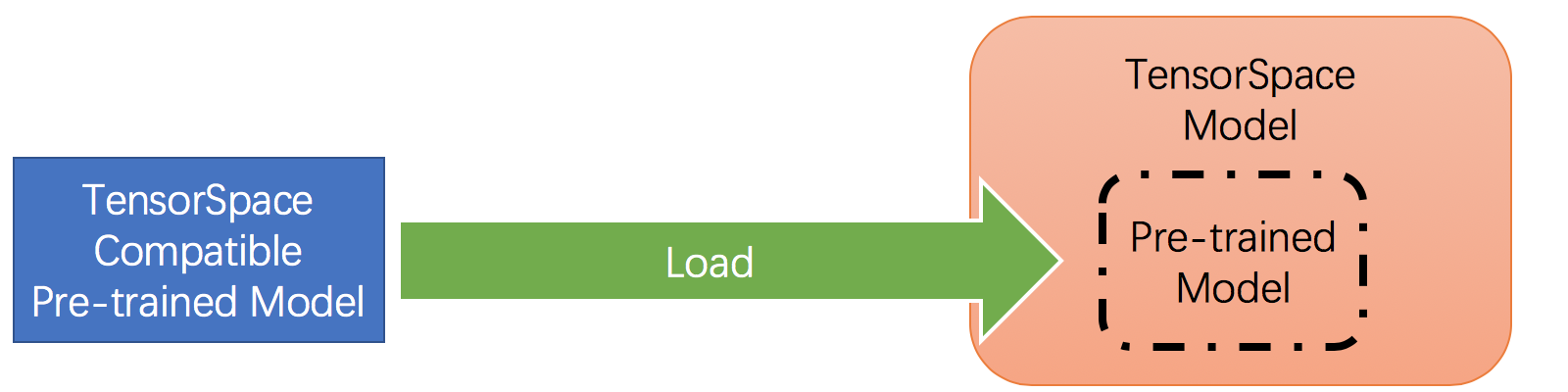
Fig. 1 - Load a preprocessed model
wb_sunnyNote:
- filter_center_focus We must preprocess the deep learning models before really loading to the TensorSpace model.
Based on different formats of deep learning models, we list different loading situations below:
Load a preprocessed Keras model
model.load( {
type: "keras",
url: '/PATH_TO_MODEL/model.json',
onProgress: function( fraction ) {
console.log( fraction );
}
onComplete: function() {
console.log( "Complete load model." );
}
} );
.type : String
- filter_center_focus Required.
- filter_center_focus Represents input ML model type.
- filter_center_focus Configure to "keras". To load as a Keras model format.
- filter_center_focus After specifying the type of network format, TensorSpace uses corresponded loader object to handle the actual loading and prediction process.
.url : String
- filter_center_focus Required.
- filter_center_focus Represents the URL to the target network structure file.
- filter_center_focus It is usually a ".json" file which represents the structure of the network.
.onProgress : void
- filter_center_focus Optional.
- filter_center_focus Progress Callback, fired periodically before loading process completed.
- filter_center_focus This callback can be used to monitor the model-loading process, for example, get "fraction" (in range [0, 1]) of model loaded at the moment as parameter.
.onComplete : void
- filter_center_focus Optional.
- filter_center_focus Callback function used to notify the complete on loading ML model.
- filter_center_focus It is designed for handling the event related to the loading complete, such as remove loading screen, console log time duration etc.
Load a preprocessed TensorFlow.js model
model.load( {
type: "tfjs",
url: '/PATH_TO_MODEL/mnist.json',
onProgress: function( fraction ) {
console.log( fraction );
}
onComplete: function() {
console.log( "Complete load model." );
}
} );
.type : String
- filter_center_focus Required.
- filter_center_focus Represents input ML model type.
- filter_center_focus Configure to "tfjs". To load as a TensorFlow.js model format.
- filter_center_focus After specifying the type of network format, TensorSpace uses corresponded loader object to handle the actual loading and prediction process.
.url : String
- filter_center_focus Required.
- filter_center_focus Represents the URL to the target network structure file.
- filter_center_focus It is usually a ".json" file which represents the structure of the network.
.onProgress : void
- filter_center_focus Optional.
- filter_center_focus Progress Callback, fired periodically before loading process completed.
- filter_center_focus This callback can be used to monitor the model-loading process, for example, get "fraction" (in range [0, 1]) of model loaded at the moment as parameter.
.onComplete : void
- filter_center_focus Optional.
- filter_center_focus Callback function used to notify the complete on loading ML model.
- filter_center_focus It is designed for handling the event related to the loading complete, such as remove loading screen, console log time duration etc.
Load a preprocessed TensorFlow model
model.load( {
type: "tensorflow",
url: "/PATH_TO_MODEL/model.json",
outputsName: [ "Maximum", "Const_1", "Maximum_1", "Const_2", "Maximum_2",
"Const_3", "Maximum_3", "Const_4", "Maximum_4", "Const_5",
"Maximum_5", "Const_6", "Const_7", "Const_8", "add_8" ],
onProgress: function( fraction ) {
console.log( fraction );
}
onComplete: function() {
console.log( "Complete load model." );
}
} );
.type : String
- filter_center_focus Required.
- filter_center_focus Represents input ML model type.
- filter_center_focus Configure to "tensorflow". To load as a TensorFlow model format.
- filter_center_focus After specifying the type of network format, TensorSpace uses corresponded loader object to handle the actual loading and prediction process.
.url : String
- filter_center_focus Required.
- filter_center_focus Represents the URL to the target ML model file.
- filter_center_focus It is usually a ".pb" file which is converted after tfjs-converter.
.outputsName : String[]
- filter_center_focus Optional.
- filter_center_focus Required if the TensorFlow model is not preprocessed for multiple outputs.
- filter_center_focus The names listed should be valid tensor names which are used for collecting the data from corresponded intermediate tensors.
- filter_center_focus Make sure the name order is the same as the one implemented in TensorSpace.
.onProgress : void
- filter_center_focus Optional.
- filter_center_focus Progress Callback, fired periodically before loading process completed.
- filter_center_focus This callback can be used to monitor the model-loading process, for example, get "fraction" (in range [0, 1]) of model loaded at the moment as parameter.
.onComplete : void
- filter_center_focus Optional.
- filter_center_focus Callback function used to notify the complete on loading ML model.
- filter_center_focus It is designed for handling the event related to the loading complete, such as remove loading screen, console log time duration etc.