3d Active function layer. The shape is not change through Activation2d layer, but values will change.
Constructor
Based on whether TensorSpace Model load a pre-trained model before
initialization, configure Layer in different ways. Checkout Layer
Configuration documentation for more information about the basic configuration rules.
〔Case 1〕If TensorSpace Model has loaded a pre-trained model before
initialization,
there is no need to configure network model related parameters.
TSP.layers.Activation3d();
〔Case 2〕If there is no pre-trained model before initialization, it is
required to configure network model related parameters.
[Method 1] Use activation
TSP.layers.Activation3d( { activation : String } );
[Method 2] Use shape
TSP.layers.Activation3d( { shape : [ Int, Int, Int ] } );
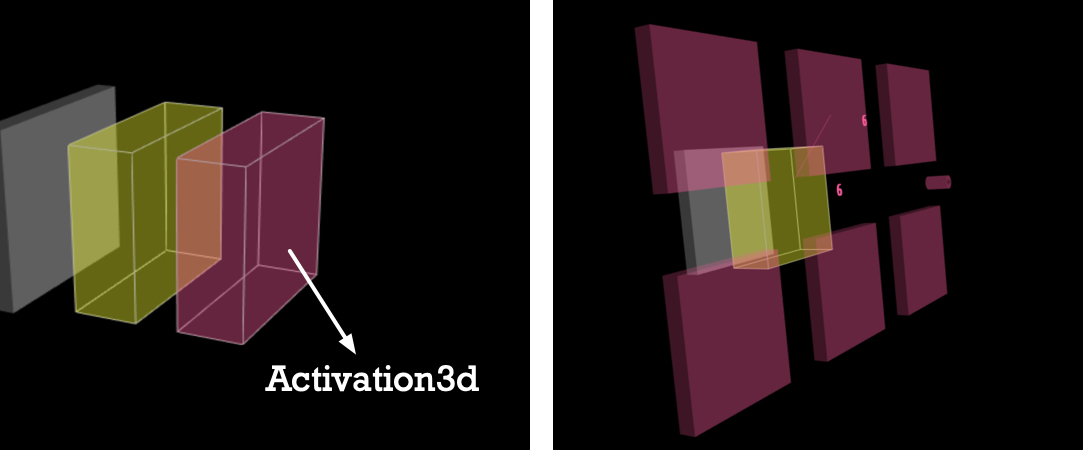
Fig. 1 - Activation3d layer close and open
Arguments
Name Tag |
Type |
Instruction |
Usage Notes and Examples |
---|---|---|---|
activation |
String | Name of active function, Network Model Related |
Options: softmax sigmoid tanh ReLU LReLU ELU |
shape |
Int[] | Output shape, Network Model Related |
For example, shape: [ 28, 28, 6 ] means the output is 3-dimensional, 6 feature maps and each one is 28 by 28. Data format is channel last. |
name |
String | Name of the layer | For example, name: "layerName" In Sequential Model: Highly recommend to add a name attribute to make it easier to get Layer object from model. In Functional Model: It is required to configure name attribute for TensorSpace Layer, and the name should be the same as the name of corresponding Layer in pre-trained model. |
color |
Color Format | Color of layer | Activation3d's default color is #FC5C9C |
closeButton |
Dict | Close button appearance control dict. More about close button | display: Bool. true[default] Show button, false Hide button ratio: Int. Times to close button's normal size, default is 1, for example, set ratio to be 2, close button will become twice the normal size |
initStatus |
String | Layer initial status. Open or Close. More about Layer initial Status | close[default]: Closed at beginning, open: Open at beginning |
animeTime |
Int | The speed of open and close animation | For example, animeTime: 2000 means the animation time will last 2 seconds. Note: Configure animeTime in a specific layer will override model's animeTime configuration. |
Properties
.inputShape : Int[]
filter_center_focusThe shape of input tensor. For
example inputShape = [ 32, 32, 3 ] represents 3 Feature maps with shape of 32
by 32.
filter_center_focusAfter model.init()
data is
available, otherwise is undefined.
.outputShape : Int[]
filter_center_focusThe shape of output tensor is
3-dimensional. 3️⃣
filter_center_focusThe shape of output tensor. For
example outputShape = [ 32, 32, 3 ] represents 3 Feature maps with shape of
32 by 32.
filter_center_focusAfter model.init()
data is
available, otherwise is undefined.
.neuralValue : Float[]
filter_center_focusThe intermediate raw data after this
layer.
filter_center_focusAfter load and model.predict() data
is available, otherwise is undefined.
.name : String
filter_center_focusThe customized name of this layer.
filter_center_focusOnce created, you can get it.
.layerType : String
filter_center_focusType of this layer, return a
constant: string Activation3d.
filter_center_focusOnce created, you can get it.
Methods
.apply( previous_layer ) : void
filter_center_focusLink this layer to previous layer.
filter_center_focusThis method can be use to construct
topology in Functional Model.
filter_center_focus See Construct Topology for more details.
.openLayer() : void
filter_center_focus Open Layer, if layer is already in
"open" status, the layer will keep open.
filter_center_focus See Layer Status for more details.
.closeLayer() : void
filter_center_focus Close Layer, if layer is already in
"close" status, the layer will keep close.
filter_center_focus See Layer Status for more details.
Examples
filter_center_focus If TensorSpace Model load a
pre-trained model before initialization, there is no need to configure network model related parameters.
let activationLayer = new TSP.layers.Activation3d( {
// Recommend Configuration. Required for TensorSpace Functional Model.
name: "Activation3d1",
// Optional Configuration.
initStatus: "open"
} );
filter_center_focus If there is no pre-trained model
before initialization, it is required to configure network model related parameters.
let activationLayer = new TSP.layers.Activation3d( {
// Required network model related Configuration.
activation: "relu",
// Recommend Configuration. Required for TensorSpace Functional Model.
name: "Activation3d2",
// Optional Configuration.
initStatus: "open"
} );
Use Case
When you add activation layer with Keras | TensorFlow | tfjs in your model the corresponding API is
Activation3d in TensorSpace.
Framework | Documentation |
---|---|
Keras | keras.activations.softmax(x, axis=-1) |
TensorFlow | tf.nn.log_softmax( logits, axis=None, name=None, dim=None ) |
TensorFlow.js | tf.layers.activation({activation: 'relu6'}) |
Source Code