Dense layer is the fully connected layer.
Constructor
Based on whether TensorSpace Model load a pre-trained model before
initialization, configure Layer in different ways. Checkout Layer
Configuration documentation for more information about the basic configuration rules.
〔Case 1〕If TensorSpace Model has loaded a pre-trained model before
initialization,
there is no need to configure network model related parameters.
TSP.layers.Dense();
〔Case 2〕If there is no pre-trained model before initialization, it is
required to configure network model related parameters.
[Method 1] Use units
TSP.layers.Dense( { units: Int } );
[Method 2] Use shape
TSP.layers.Dense( { shape : [ Int ] } );
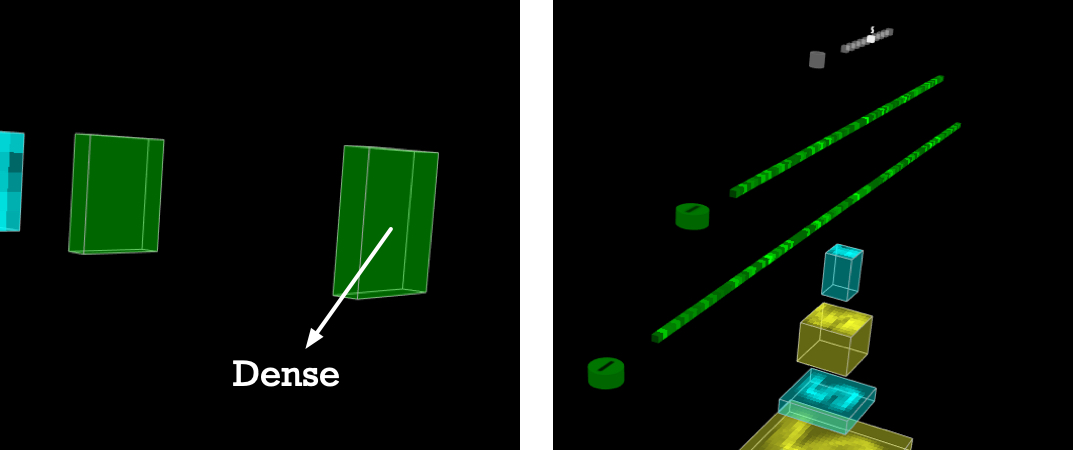
Fig. 1 - Dense layer close and open
Arguments
Name Tag |
Type |
Instruction |
Usage Notes and Examples |
---|---|---|---|
units |
Int | Amount of nodes of FC layer, Network Model Related |
For example, units = 100 means there are 100 neural in the fully connect layer |
shape |
Int[] | Output shape, Network Model Related |
For example, shape: [ 100 ] means the output is 1-dimensional array by 100 |
paging |
Bool | Enable Pagination. More about paging | Since the long 1D layer could make the model hard to visualize, we use paging. true Enable; false [Default] Disable |
segmentLength |
Int | Amount of nodes in one page, only take effect when "paging" is enabled | 200[Default] 200 nodes in each page |
segmentIndex |
Int | The initial start index of first page, only take effect when "paging" is enabled | 0[Default] |
overview |
Bool | Look layer Text from different angle. More about overview | false[Default] |
name |
String | Name of the layer | For example, name: "layerName" In Sequential Model: Highly recommend to add a name attribute to make it easier to get Layer object from model. In Functional Model: It is required to configure name attribute for TensorSpace Layer, and the name should be the same as the name of corresponding Layer in pre-trained model. |
color |
Color Format | Color of layer | Dense's default color is light green #00FF00 |
closeButton |
Dict | Close button appearance control dict. More about close button | display: Bool. true[default] Show button, false Hide button ratio: Int. Times to close button's normal size, default is 1, for example, set ratio to be 2, close button will become twice the normal size |
initStatus |
String | Layer initial status. Open or Close. More about Layer initial Status | close[default]: Closed at beginning, open: Open at beginning |
animeTime |
Int | The speed of open and close animation | For example, animeTime: 2000 means the animation time will last 2 seconds. Note: Configure animeTime in a specific layer will override model's animeTime configuration. |
Properties
.inputShape : Int[]
filter_center_focusThe shape of input tensor, for
example inputShape = [ 28, 28, 3 ] represents 3 feature maps and each
one is 28 by 28.
filter_center_focusAfter model.init()
data is
available, otherwise is undefined.
.outputShape : Int[]
filter_center_focusThe shape of output tensor is
1-dimensional. 1️⃣
filter_center_focusThe shape of output tensor. For
example outputShape = [ 1000 ] represents the output of this layer
has 1000 outputs.
filter_center_focusAfter model.init()
data is
available, otherwise is undefined.
.neuralValue : Float[]
filter_center_focusThe intermediate raw data after this
layer.
filter_center_focusAfter load and model.predict() data
is available, otherwise is undefined.
.name : String
filter_center_focusThe customized name of this layer.
filter_center_focusOnce created, you can get it.
.layerType : String
filter_center_focusType of this layer, return a
constant: string Dense.
filter_center_focusOnce created, you can get it.
Methods
.apply( previous_layer ) : void
filter_center_focusLink this layer to previous layer.
filter_center_focusThis method can be use to construct
topology in Functional Model.
filter_center_focus See Construct Topology for more details.
.openLayer() : void
filter_center_focus Open Layer, if layer is already in
"open" status, the layer will keep open.
filter_center_focus See Layer Status for more details.
.closeLayer() : void
filter_center_focus Close Layer, if layer is already in
"close" status, the layer will keep close.
filter_center_focus See Layer Status for more details.
Examples
filter_center_focus If TensorSpace Model load a
pre-trained model before initialization, there is no need to configure network model related parameters.
let denseLayer = new TSP.layers.Dense( {
// Recommend Configuration. Required for TensorSpace Functional Model.
name: "Dense1",
// Optional Configuration.
initStatus: "open"
} );
filter_center_focus If there is no pre-trained model
before initialization, it is required to configure network model related parameters.
let denseLayer = new TSP.layers.Dense( {
// Required network model related Configuration.
units: 1000,
// Recommend Configuration. Required for TensorSpace Functional Model.
name: "Dense2",
// Optional Configuration.
initStatus: "open"
} );
Use Case
When you add fully connect layer with Keras | TensorFlow | tfjs in your model the corresponding API is
Dense in TensorSpace.
Framework | Documentation |
---|---|
Keras | keras.layers.Dense(units) |
TensorFlow | tf.layers.dense(inputs, units) |
TensorFlow.js | tf.layers.dense (config) |
Source Code