"Add" is constructed as a "factory" to complete the "add" operation.
To apply the "Add" function, it is required that the shapes of all input layers are the same
(otherwise, we can't perform the operation). The "factory" returns a
merged layer instance as the output.
Factory style
layerAddResult = TSP.layers.Add( [layer1, layer2, ...], { configs } )
filter_center_focus The first parameter is a list of layer objects.
filter_center_focus The second parameter is the
configurations of the output merged layer object.
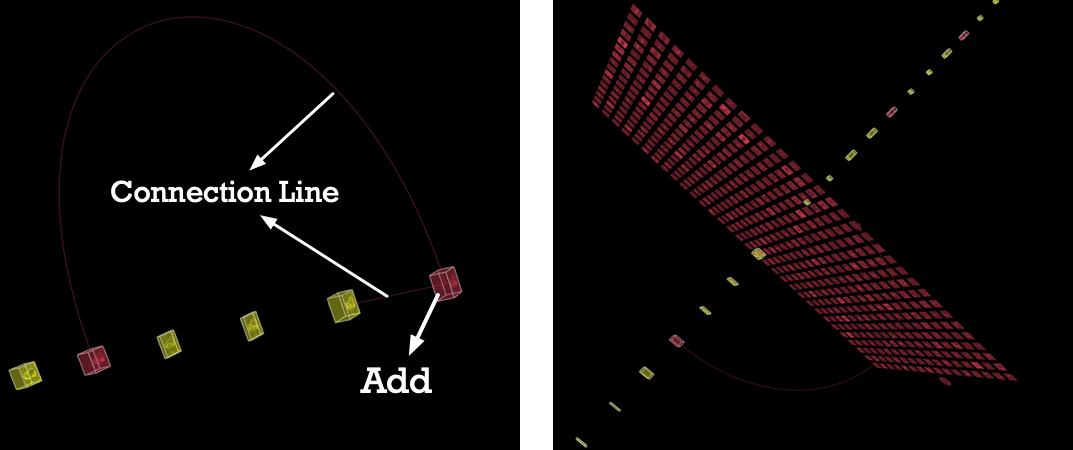
Fig. 1 - Add layer close and open
Configuration
Name Tag |
Type |
Instruction |
Usage Notes and Examples |
---|---|---|---|
name |
String | Name of the layer | For example, name: "layerName" In Sequential Model: Highly recommend to add a name attribute to make it easier to get Layer object from model. In Functional Model: It is required to configure name attribute for TensorSpace Layer, and the name should be the same as the name of corresponding Layer in pre-trained model. |
color |
Color Format | Color of layer | Add's default color is red #E23E57 |
closeButton |
Dict | Close button appearance control dict. More about close button | display: Bool. true[default] Show button, false Hide button ratio: Int. Times to close button's normal size, default is 1, for example, set ratio to be 2, close button will become twice the normal size |
initStatus |
String | Layer initial status. Open or Close. More about Layer initial Status | close[default]: Closed at beginning, open: Open at beginning |
animeTime |
Int | The speed of open and close animation | For example, animeTime: 2000 means the animation time will last 2 seconds. Note: Configure animeTime in a specific layer will override model's animeTime configuration. |
Properties
.outputShape : Int[]
filter_center_focusThe shape of the output tensor is
3-dimensional. 3️⃣
filter_center_focusThe dataFormat is "channel last".
For example, outputShape = [ 32, 32, 4 ] represents the output through this
layer has 4 feature maps and each one is 32 by 32.
filter_center_focusAfter model.init()
data is available, otherwise is undefined.
.neuralValue : Float[]
filter_center_focusThe intermediate raw data after this
layer.
filter_center_focusAfter load and model.predict(), the data are available. Otherwise is undefined.
.name : String
filter_center_focusThe customized name of this layer.
filter_center_focusOnce created, you can get it.
.layerType : String
filter_center_focusType of this layer, return a
constant: string Add1d, Add2d, or Add3d. This type can identify the dimension
of
input layers
filter_center_focusOnce created, you can get it.
Methods
.openLayer() : void
filter_center_focus Open Layer, if layer is already in
"open" status, the layer will keep open.
filter_center_focus See Layer Status for more details.
.closeLayer() : void
filter_center_focus Close Layer, if layer is already in
"close" status, the layer will keep close.
filter_center_focus See Layer Status for more details.
Examples
filter_center_focus Add two TensorSpace Layers, get Add
Layer instance:
let addLayer = TSP.layers.Add(
[ layer1, layer2 ],
{
// Recommend Configuration. Required for TensorSpace Functional Model.
name: "AddLayer",
// Optional Configuration.
animeTime: 4000,
initStatus: "open"
}
);
Use Case
When you add add operation layer with Keras | TensorFlow | tfjs in your model the corresponding API is Add
in TensorSpace.
Framework | Documentation |
---|---|
Keras | keras.layers.Add() |
TensorFlow | tf.keras.layers.add( inputs, **kwargs ) |
TensorFlow.js | tf.layers.add (config) |
Source Code