YoloGrid layer is specifically design for YOLO
model which is used to determine the center of the rectangle to mark the position of the object.
The main idea of YOLO is to divide the image into regions and predicts
bounding boxes and probabilities for each region. This YoloGird is
interactive with this feature map and each region. You can click at this layer to draw the rectangle at next
layer: ObjectDetection which can draw a rectangle on the image.
The shape of the feature map will be calculate automatically according to inputShape.
Constructor
Use anchors classLabelList configuration
related to YOLO model and a callback function named onCeilClicked
to construct.
let yoloGrid = new TSP.layers.YoloGrid( {
anchors: [ 1.08, 1.19, 3.42, 4.41, 6.63, 11.38, 9.42, 5.11, 16.62, 10.52 ],
//voc class label name list
classLabelList: [ "aeroplane", "bicycle", "bird", "boat", "bottle",
"bus", "car", "cat", "chair", "cow",
"diningtable", "dog", "horse", "motorbike", "person",
"pottedplant", "sheep", "sofa", "train", "tvmonitor" ],
onCeilClicked: onYoloCeilClicked
} );
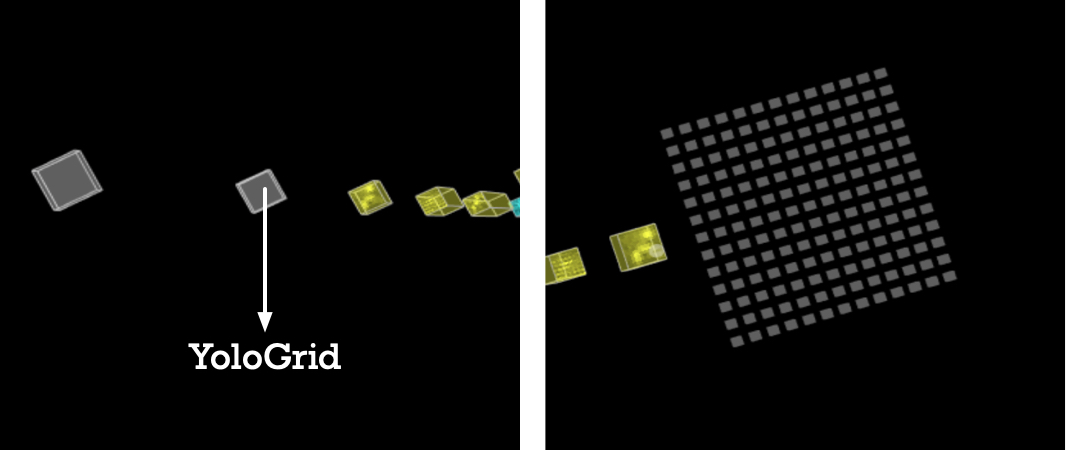
Fig. 1 - YoloGrid layer close and open
Arguments
Name Tag |
Type |
Instruction |
Usage Notes and Examples |
---|---|---|---|
anchors |
Float[] | Previous Probability of anchors | Check it at cfg file of darknet configuration, also can be found at our examples. |
classLabelList |
String[] | Output class label names | Depend on the data set: VOC has 20 classes,COCO has 80,ImageNet has 1000 |
onCeilClicked |
Function | Fired when ceil is clicked, return data array store in array | Check out this example for more information ceilData: Return the output data. rectList: The rectangle coordinate to present the object. |
name |
String | Name of the layer | For example, name: "layerName" In Sequential Model: Highly recommend to add a name attribute to make it easier to get Layer object from model. In Functional Model: It is required to configure name attribute for TensorSpace Layer, and the name should be the same as the name of corresponding Layer in pre-trained model. |
color |
Color Format | Color of layer | YoloGrid's default color is #EEEEEE |
closeButton |
Dict | Close button appearance control dict. More about close button | display: Bool. true[default] Show button, false Hide button ratio: Int. Times to close button's normal size, default is 1, for example, set ratio to be 2, close button will become twice the normal size |
initStatus |
String | Layer initial status. Open or Close. More about Layer initial Status | close[default]: Closed at beginning, open: Open at beginning |
animeTime |
Int | The speed of open and close animation | For example, animeTime: 2000 means the animation time will last 2 seconds. Note: Configure animeTime in a specific layer will override model's animeTime configuration. |
Properties
.name : String
filter_center_focusThe customized name of this layer.
filter_center_focusOnce created, you can get it.
.layerType : String
filter_center_focusType of this layer, return a
constant: string YoloGrid.
filter_center_focusOnce created, you can get it.
Methods
.apply( previous_layer ) : void
filter_center_focusLink this layer to previous layer.
filter_center_focusThis method can be use to construct
topology in Functional Model.
filter_center_focus See Construct Topology for more details.
.openLayer() : void
filter_center_focus Open Layer, if layer is already in
"open" status, the layer will keep open.
filter_center_focus See Layer Status for more details.
.closeLayer() : void
filter_center_focus Close Layer, if layer is already in
"close" status, the layer will keep close.
filter_center_focus See Layer Status for more details.
Examples
filter_center_focus Declare an instance of YoloGrid
let yoloGrid = new TSP.layers.YoloGrid( {
anchors: [ 1.08, 1.19, 3.42, 4.41, 6.63, 11.38, 9.42, 5.11, 16.62, 10.52 ],
//voc class label name list
classLabelList: [ "aeroplane", "bicycle", "bird", "boat", "bottle",
"bus", "car", "cat", "chair", "cow",
"diningtable", "dog", "horse", "motorbike", "person",
"pottedplant", "sheep", "sofa", "train", "tvmonitor" ],
onCeilClicked: onYoloCeilClicked
} );
function onYoloCeilClicked( ceilData, rectList ) {
// some operation with ceilData and rectList
}
Source Code